mining algorithm
TERA HASH
- Introduction
- Algorithm
- Small calculations
- JS code
Introduction
The purpose of this algorithm is to equalize the people who are mining on a CPU and the people who are mining on a GPU. To achieve this, we suggest to use memory, but unlike other similar algorithms (such as Ethash) in our algorithm, memory does not slow down the GPU, but accelerates a CPU. This can be done by using not an integer nonce when selecting a hash, but a certain value that is time - consuming to calculate-for example, calculated by the sha3 algorithm and allowing this value to be used for enumeration in a wide range of block hash calculations. Thus, it is more profitable to store these values in memory for selection than to recalculate them. This benefit should be maintained even if the speed of computing resources will increase by 1000 times.
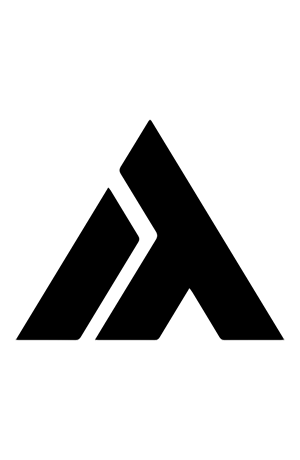
Algorithm
The input is a 32-byte hash of the current block CurrentDataHash, you need to find a Nonce (integer), so that the result is a suitable hash of the block with the maximum value of initial zeros.
Restriction:
1. The search should be optimized for memory usage - for protection from GPU mining
2. The check should be performed with a minimum amount of memory and be fast-about the speed of sha3 calculation
Procedure of settlements:
1. Calculated HashNonce= sha3(PrevHashN , Nonce)
2. The Resulting Hash = SimpleMesh(HashTemp,CurrentDataHash)
PrevHashN is a 32-byte hash of some previous block, different from the current block on NDelta (the maximum depth is limited to a certain number, for example 1000 blocks)
Nonce-a number to iterate through values from 0 to max of an integer
SimpleMesh () is a function of the rapid mixing (non-cryptographic). It must satisfy the conditions:
1. The conservation of entropy
2. High speed-1000 times faster than the function calculation speed in step 1 (sha3 in this example).
3. It should be good to mix the data - to prevent a quick search, i.e. guarantee busting of HashNonce
After searching for the most satisfying hash, the blockchain is written: CurrentDataHash, Nonce, NDelta - which quickly restores the block hash
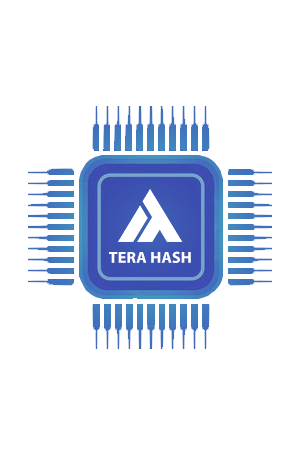
Small calculations
Small calculations:
● GPU GTX 1060 for 1 second will create and compare the order of 0.5 billion hashes.
● Computer: using the memory DDR4-2133 will have to have speed channel is 17Gb/s and therefore will be able to compare also the order of 0.5 billion hashes
Miner must prove that the base hash has not changed. He needs to find a nonce two:
One that fits the current hash of the block
Different to the previous block hash
The main calculation formula:
Hash = XOR(DataHash, HNonce1)
Hash2 = XOR(PrevHash,HNonce2)
Power=min(Power(Hash),Power(Hash2))
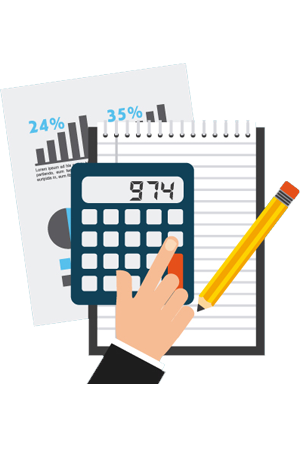
JS code:
function GetHash(PrevHash,BlockHash,Miner,BlockNum,Nonce0,
Nonce1,Nonce2,DeltaNum1,DeltaNum2)
{
if(DeltaNum1>DELTA_LONG_MINING || DeltaNum2>DELTA_LONG_MINING)
return undefined;
//calculate the hashes, which will look similar HashNonce
var HashBase=sha3(PrevHash);
var HashCurrent=GetHashFromNum2(BlockHash,Miner,Nonce0);
//Hash-Nonce
var HashNonce1=GetHashFromNum3(BlockNum-DeltaNum1,Miner,Nonce1);
var HashNonce2=GetHashFromNum3(BlockNum-DeltaNum2,Miner,Nonce2);
//XOR
var Hash1=XORArr(HashBase,HashNonce1);
var Hash2=XORArr(HashCurrent,HashNonce2);
//choose the least POW
var Ret={Hash:Hash2};
if(CompareArr(Ret.Hash1,Ret.Hash2)>0)
{
Ret.PowHash=Hash1;
}
else
{
Ret.PowHash=Hash2;
}
Ret.Hash=sha3arr2(Hash1,Hash2);
return Ret;
}
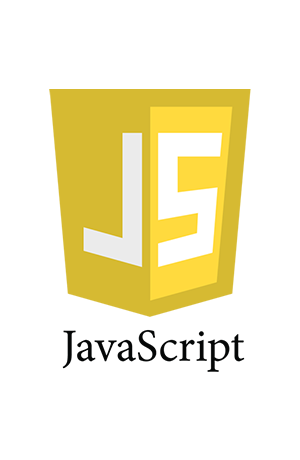
Manuals
Start Mining
Build From Source
- Attention!
- Windows
- CentOS 7
- UBUNTU 18.4
Attention!
- After the installation, enter the address ofyour server in a browser. Example: 12.34.56.78:8080
- For mining You must have a static (public) IP address and an open port.
- We recommend not storing private keys on remote servers.
- We recommend putting an additional password on the private key ("Set password" button) - in this case the private key will be stored in file in encrypted form.
- If you do not specify the http password for full-node, you can only access from the local address 127.0.0.1:8080 and only within 10 minutes after the launch of the node
- For remote access to the node only from the specified computer (white IP) set the HTTP_IP_CONNECT constant (for example: "HTTP_IP_CONNECT": "122.22.33.11")
- When installing, pay attention to the secp256k1 cryptographic library. There should be no errors when compiling it (with command: npm install)
- If you have the auto-update constant enabled ("USE_AUTO_UPDATE": 1), the update is performed automatically. If off, then you need to load it manually and restart the node (see section below).
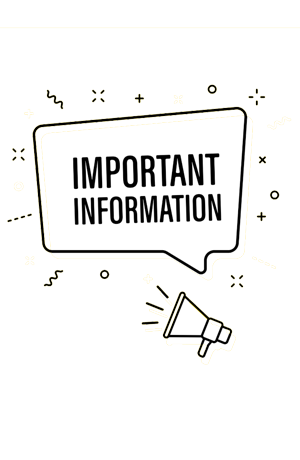
Windows
- Download and install Nodejs
- Download and install git
- Then run the commands (in program: cmd or PowerShell):
- cd ..\..\..\
- git clone https://gitlab.com/terafoundation/tera2.git wallet
- npm install --global --production windows-build-tools
- npm install -g node-gyp
- cd wallet/Source
- npm install
- node set httpport:8080 password:password_no_spaces
- run-node.bat
Before starting the node, we recommend to download a backup of the blockchain (zip size 10 GB) Here:
Unpack the archive and put the DB folder in the wallet's DATA folder (with full replacement).
Launch the node with the command:
- run-node.bat
If you want to run the wallet as a background process, then instead of the last command (run-node.bat), do the following:
- npm install pm2 -g
- pm2 start run-node.js
Opening Port
- netsh advfirewall firewall add rule name="Open 30000 port" protocol=TCP localport=30000 action=allow dir=IN
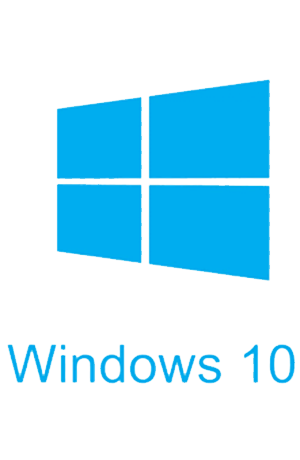
CentOS 7
Run Commands:
- yum install install unzip
- yum install -y git
- curl --silent --location https://rpm.nodesource.com/setup_8.x | sudo bash -
- yum install -y nodejs
- yum install gcc gcc-c++
- npm install pm2 -g
- git clone https://gitlab.com/terafoundation/tera2.git wallet
- cd ~/wallet/Source
- npm install
- node set httpport:8080 password:password_no_spaces
Open Ports (all):
- systemctl stop firewalld
- systemctl disable firewalld
Before starting the node, we recommend downloading a backup of the blockchain (zip size 10 GB)
- cd ~/wallet/DATA
- wget https://terawallet.org/files/jinn-db.zip
- unzip -o jinn-db.zip
Start Node:
- cd ~/wallet/Source
- pm2 start run-node.js
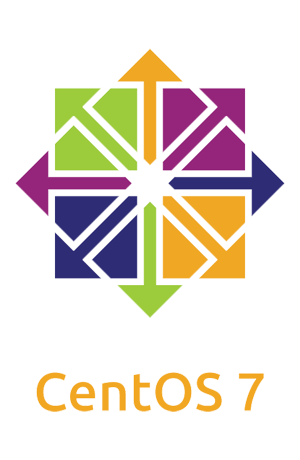
UBUNTU 18.4
Run Commands:
- apt-get install unzip
- apt-get install -y git
- apt-get install -y nodejs
- apt-get install -y npm
- npm install pm2 -g
- git clone https://gitlab.com/terafoundation/tera2.git wallet
- apt install build-essential
- apt group install "Development Tools"
- cd ~/wallet/Source
- npm install
- node set httpport:8080 password:password_no_spaces
Open Ports:
- sudo ufw allow 30000/tcp
- sudo ufw allow 8080/tcp
- sudo ufw allow 80/tcp
Before starting the node, we recommend downloading a backup of the blockchain (zip size 10 GB)
- cd ~/wallet/DATA
- wget https://terawallet.org/files/jinn-db.zip
- unzip -o jinn-db.zip
Start Node:
- cd ~/wallet/Source
- pm2 start run-node.js
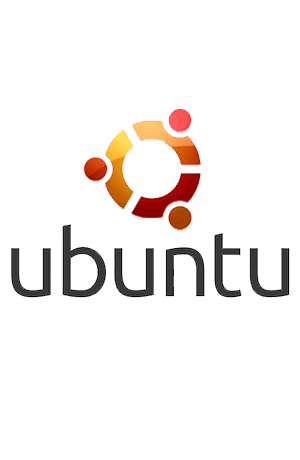
DOCKER
CONSTANTS
Description of constants (from version 2360):
- //IP-PROTOCOL
- //CLUSTERS
- //STATISTICS
- //Information display
- //HTTP access to the full-nodes
- //MINING:
- //HTTP access to the light node
- //parameters:
- //ETC
//IP-PROTOCOL
"IP_VERSION": 4, | //version of ip Protocol, valid values are 4 or 6 |
"JINN_IP": "10.20.30.40", | //the public IP address of the node, if not specified, is determined automatically |
"JINN_PORT": 30000, | //port |
"AUTODETECT_IP": 0, | //ip address detection mode (i.e., the value of the JINN_IP constant is reset at startup) |
"CLIENT_MODE": 0 | //mode works only in client mode without a possibility to accept incoming connections |
//CLUSTERS
"COMMON_KEY": "Secret1", | //the total key for the trusted nodes (the nodes not to ban each other) |
"NODES_NAME": "Node001", | //the name of the trusted node that appears on the NETWORK page |
"CLUSTER_HOT_ONLY", | //enable communication only with the nodes of the cluster |
//STATISTICS
"STAT_MODE": 1, | //enable statistics-see Counters, Monitor pages |
"MAX_STAT_PERIOD": 600, | //statistics period |
//Information display
"LOG_LEVEL":2, | //logging details, the higher the number, the more messages |
"COUNT_VIEW_ROWS": 20, | //number of rows per page - displayed in all tables with pagination |
"ALL_VIEW_ROWS": 1, | //view all rows of blockchain tables, even if they are blacklisted (for example, DAPs misleading) |
"START_HISTORY": 16, | //the account number from which the transaction history is recorded |
//HTTP access to the full-nodes wallet
"HTTP_PORT_NUMBER": 8000, | //http access port |
"HTTP_PORT_PASSWORD": "SecretWord", | //password |
"HTTP_IP_CONNECT": "123.111.222.233, 123.11.22.223", | //enable IP address filter for remote access |
"WALLET_NAME": "=Node1=", | //the name of the wallet displayed on the tab in the browser |
//MINING:
"USE_MINING": true, | //enable mining |
"MINING_ACCOUNT": 0, | //number of the account that receives the reward for the found block |
"MINING_START_TIME": "6:30", | //mining start time during the day in the format hh:mm: ss time in UTC (mining must be enabled by the constant USE_MINING), if the value is not set then mining is performed all the time |
"MINING_PERIOD_TIME": "16:30", | //the period of mining since the beginning of the format hh: mm:ss |
"POW_MAX_PERCENT": "70", | //max CPU usage as a percentage of each mining process |
"COUNT_MINING_CPU": 0, | //number of processes to run during mining |
"SIZE_MINING_MEMORY": 20000000, | //the total number of memory allocated for mining on all processes (in bytes) |
"POW_RUN_COUNT": 5000, | //the number of hashes (hash1, hash2) calculated at each mining iteration in each process (each iteration is started once in 1 MS - but within this second the POW_MAX_PERCENT parameter is taken) |
//HTTP access to the light node, API1 and API2
"USE_API_WALLET": 1, | //enabling light (web) client support |
"USE_API_V1": 1, | //enabling API1 |
"MAX_TX_FROM_WEB_IP": 20, | //limit the number of tx (every 10 minutes) |
"USE_HARD_API_V2": 0, | //enabling API2 node support is not recommended for public access |
//parameters:
"HTTP_HOSTING_PORT": 80, | //web port number |
"HTTPS_HOSTING_DOMAIN": "", | //domain name |
"HTTP_MAX_COUNT_ROWS": 20, | //limit on the number of rows of data requests |
"HTTP_START_PAGE": "", | //home page (default web wallet) |
"HTTP_CACHE_LONG": 10000, | //page cache time (sec) |
"HTTP_USE_ZIP": 0, | //using the page archiving |
"WEB_LOG": 0, | //enabling logging |
//ETC
"USE_AUTO_UPDATE": 1, | //using online auto-updates |
"JINN_MAX_MEMORY_USE": 400, | //memory limit that is used for the blockchain database cache in MB |
"RESTART_PERIOD_SEC": 0, | //auto restart nodes after a specified period in seconds |
"NOT_RUN": 0, | //disabling the node |
"DELTA_CURRENT_TIME": -50, | //time deviation relative to Tera network (set automatically) |
"LOG_LEVEL":2, | //logging details, the higher the number, the more messages |
## Note:
* Constants are stored in JSON format
* In logical constants, such as enabling or disabling something, we recommend using the number 0 or 1
* Any constant can be changed from the command line as follows (run inside the ~/wallet/Source directory): node set NAME=Value
* After changing the constants restart the node
Top Miners
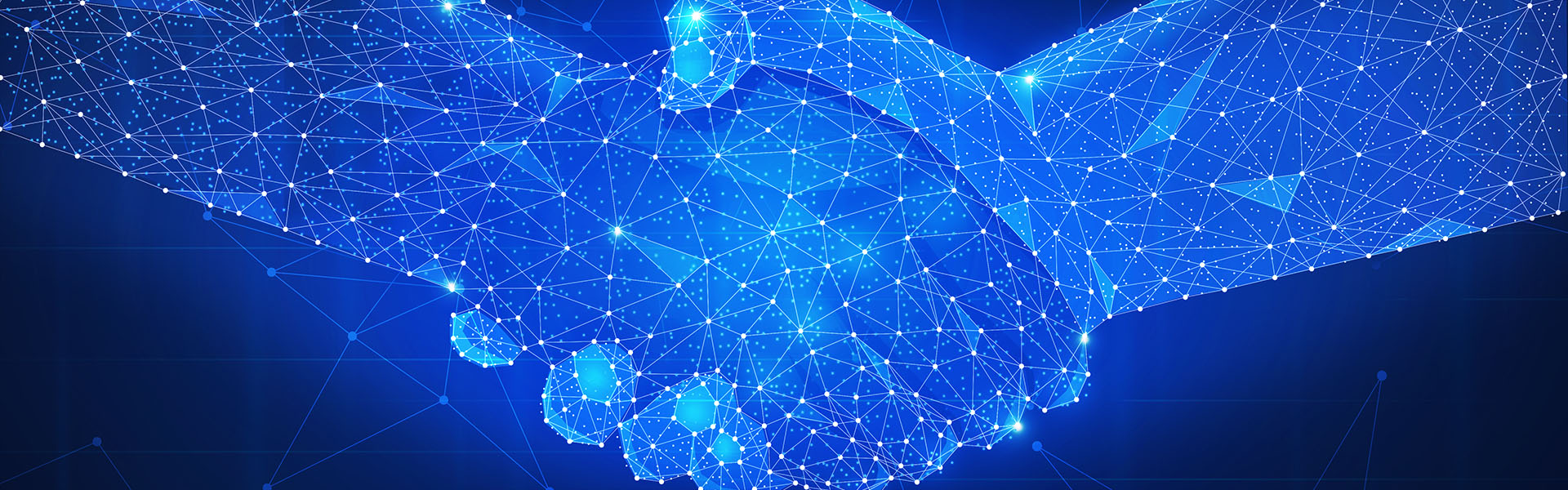